Hi All,
I'm currently a newbie to WebDev and JavaScript.
I'm Attempting an exercise involving the concept of localStorage in JS.
The exercise involves taking user input of their favourite songs and adding it to localStorage and displaying the data on the DOM.
The requirements are to add a song and display this individually on the page, and then delete an instance of each song from the localStorage and the subsequently from the webpage.
I'm having challenges actually deleting the song from the localStorage -- if I click on the delete button -- it should delete that instance of the song from the localStorage array and the DOM BUT it actually deletes 2 or more objects from the arrays on of Songs I have in localStorage.
I've tried everything I could think of and would love advice on how to solve this issue. I've shared the JS code and user interface for your perusal.
Thanks in advance
`
JAVASCRIPT CODE
//Retrieving all the elements
let artistName = document.getElementById("artistName");
let songTitle = document.getElementById("title");
let albumTitle = document.getElementById("album");
let yearReleased = document.getElementById("rel-year");
//Initiated an empty array to store all songs
let songArray = [];
function pageLoad() {
if (localStorage.getItem("hasCodeRunBefore") === null) {
localStorage.setItem("songs", JSON.stringify(songArray));
localStorage.setItem("hasCodeRunBefore", true);
} else {
//Using a forEach loop to loop through the songArray to populate the song data on the webpage
let songContainer = document.getElementById("song-display-container");
songContainer.innerHTML = "";
songArray = JSON.parse(localStorage.getItem("songs"));
songArray.forEach(function (song, index) {
let songDisplay = document.createElement("div");
songDisplay.setAttribute("id", "song-display");
songDisplay.innerHTML =
;
<h2>Song: ${song.songTitle}</h2>
<ul>
<li>Artist: ${song.artist}</li>
<li>Album: ${song.albumTitle}</li>
<li>Release Year: ${song.releaseYear}</li>
<li>Genre: ${song.musicGenre}</li>
</ul>
songContainer.appendChild(songDisplay);
//Code to delete the song from DOM and localStorage
let btn = document.createElement("button");
btn.setAttribute("class", "btn btn-primary");
btn.innerHTML = "Delete";
songDisplay.appendChild(btn);
btn.addEventListener("click", function (event) {
//Deleting song entry from the DOM
let songItem = event.target;
songItem.parentElement.remove();
//Deleting song entry from local storage
songArray.splice(index);
localStorage.setItem("songs", JSON.stringify(songArray));
});
});
}
}
/ADDING A NEW SONG TO [SONG ARRAY] AND LOCAL STORAGE/
//Used the constructor function to create the Song class with properties.
function Song(aName, title, album, relYear, genre) {
this.artist = aName;
this.songTitle = title;
this.albumTitle = album;
this.releaseYear = relYear;
this.musicGenre = genre;
}
//Function to add to new song object to the songArray and localStorage.
function addSong() {
let musicGenre = document.getElementById("genre-select");
songArray = JSON.parse(localStorage.getItem("songs"));
let newSong = new Song(
artistName.value,
songTitle.value,
albumTitle.value,
yearReleased.value,
musicGenre.value
);
songArray.push(newSong);
localStorage.setItem("songs", JSON.stringify(songArray));
pageLoad();
}
//CLEAR LOCAL STORAGE
function clearLS() {
localStorage.clear();
}
`
Top comments (3)
Your issue might be stemming from the event binding when deleting ensure the event listeners correspond correctly to the targeted element for deletion in both the DOM and the localStorage.
Best Inground Pool Remodeling in Sylmar California
JavaScript's localStorage is a feature that allows web applications to store data locally within the user's web browser. It provides a simple key-value storage mechanism and is widely used for storing small amounts of data persistently. Here's a brief overview of how to use localStorage in JavaScript:
Storing Data:
To store data in localStorage, you can use the setItem() method. It takes two parameters: the key (as a string) and the value (also as a string).
javascript
Copy code
localStorage.setItem('key', 'value');
Retrieving Data through umn webmail:
To retrieve data from localStorage, you can use the getItem() method. It takes the key as a parameter and returns the corresponding value.
This is the image of the simple UI
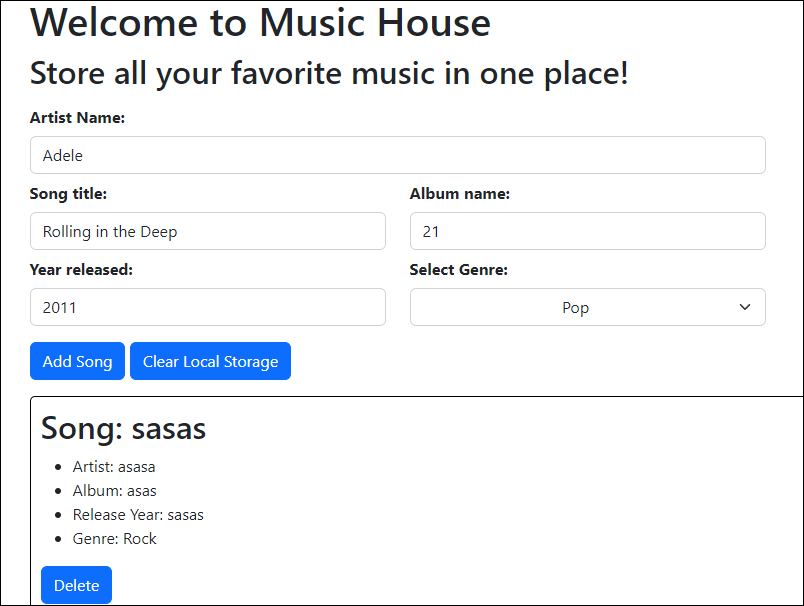